Image Blurring in NextJS
Dec 26, 2024
1 min read
Image blurring improves user experience during slow loading times by providing a placeholder while the full image loads. This helps optimize the Largest Contentful Paint (LCP) metric.
We'll use the Plaiceholder package to create Low Quality Image Placeholders (LQIP). Here's how to set it up:
Installation:
npm install plaiceholder @plaiceholder/next
Configure Next.js:
// next.config.mjs
import withPlaiceholder from "@plaiceholder/next";
const config = {
// your Next.js config
};
export default withPlaiceholder(config);
Plaiceholder offers several methods for creating placeholders:
- Color: Extracts dominant color
- CSS (~600B): Creates linear-gradient placeholders
- SVG (~1.2KB): Generates low-res SVG placeholders
- Base64 (~300B): Produces low-res encoded images
Here's a utility function to generate Base64 placeholders:
import { getPlaiceholder } from "plaiceholder";
export const getPlaiceholderImage = async (imageUrl: string) => {
const buffer = await fetch(imageUrl).then((res) => res.arrayBuffer());
const { ...plaiceholder } = await getPlaiceholder(Buffer.from(buffer));
return {
...plaiceholder,
img: { src: imageUrl },
};
};
Implementation example:
const WorkCard = async ({ work }: WorkCardProps) => {
const { img, base64 } = await getPlaiceholderImage(work.image?.asset?._ref);
return (
<div className='relative h-40 w-full overflow-hidden rounded-lg'>
<Image
src={img.src}
alt={work.title}
fill
placeholder='blur'
blurDataURL={base64}
className='object-cover rounded-lg overflow-hidden ease-in-out'
/>
</div>
);
};
Note: The package uses Sharp under the hood. While serverless costs are minimal, consider implementing revalidation to optimize performance.
Blurred Images Example:
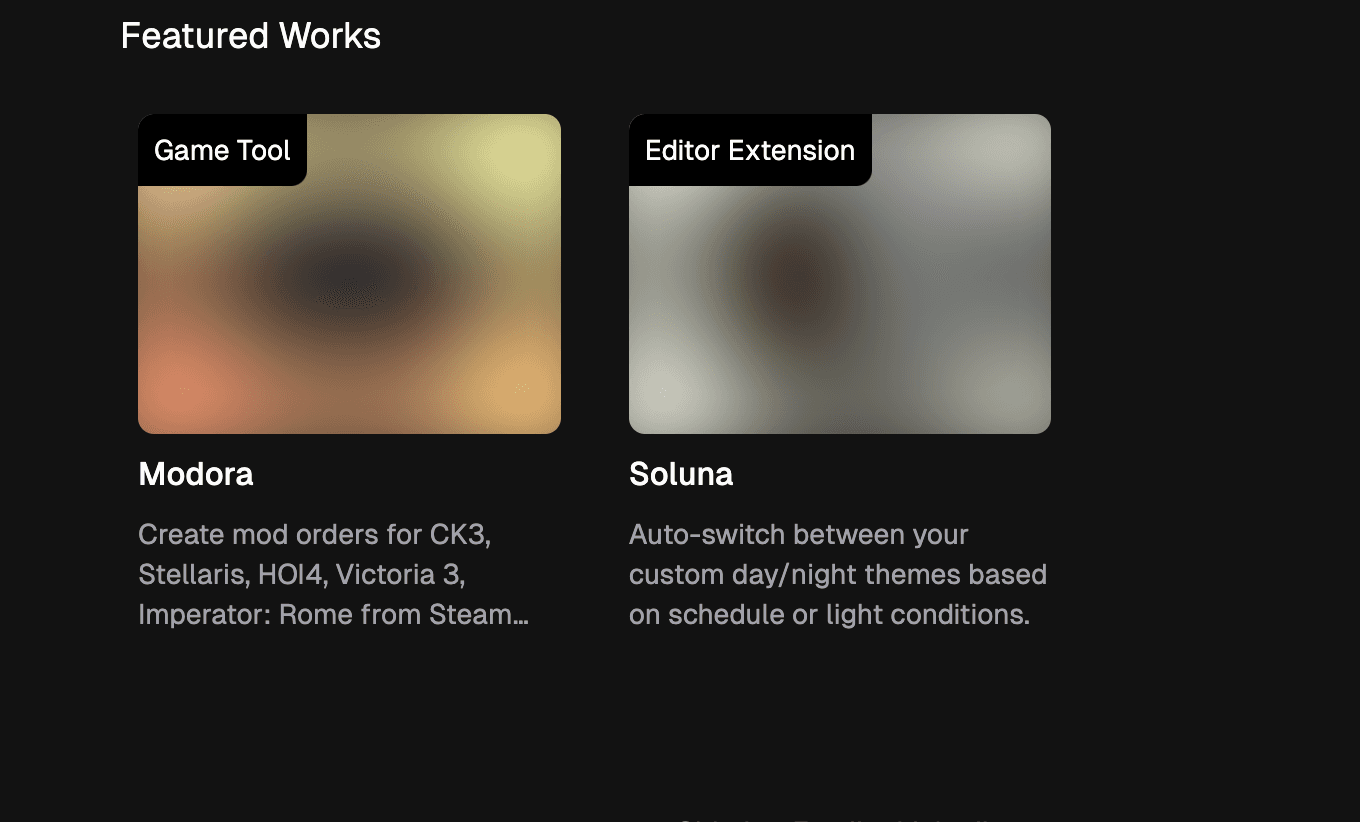